It has been almost a week already! Hope you have fiddled more with the project from
last week and the
week before. Our new project has only one sprite and a very short script. Yet it illustrates interesting ideas on simulating falling objects and using the audio input to control a sprite. We will need a microphone for this project.
This is what we will create:
Just a few simple steps:
Step 1: Setup stage, sprite and variables
The Stage is really simple for this one. We will just whip up the Paint Editor and put in a few strokes of blue for our "water".
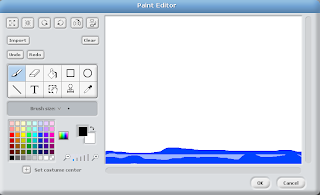 |
Painting "water" on the Stage |
In this project we will use two variables to help program the movement of the ball. To create a variable, first click on the ball sprite (you would have to add it first, of course). Then go to the Block Palette and click on the "Variables" block group. Under the group, you will see the "Make a variable" button.
 |
The "Make a variable" button under the "Variables" block group |
Click on the "Make a variable" button and a you see a small popup. That's for us to name the variable. Select "For this sprite only" option, and name the variable
old y:
 |
Creating a variable for the ball sprite |
Once we hit "OK", we will get a variable named
old y whose value can be read by the ball sprite only. Create one more variable and call it
y velocity. This is what you should see once done:
 |
Our two variables |
What is left of the setup now is for us to set (or in programmer speak, "initialize" our ball position and the value of the
y velocity variable. This ensures that every time we start the program by clicking the green flag, the ball is positioned at the right place and
y velocity is reset to 0.
 |
Initializing ball position and the "y velocity" variable
|
Step 2: Program ball movement
How are we going to program the ball movement? Before we get our hands dirty, let's talk about how a ball really moves in the real world. If we hold a ball still in the air, it has zero velocity because it does not move. The ball has a certain position - it could be say, 100m off the ground.
Now what happens when we release our grip on the ball? At the very instant we release it, the only force working on the ball is gravity. The ball now picks up velocity, and moves faster and faster towards the ground.
Let's rewind this scene and play it very, very slowing in our heads. Imagine the movement of the ball at
every second while it falls. Suppose the ball falls 0.1m after
one second. Its position after that one second is now 99.9m off the ground. Since it fell 0.1m in one second, its velocity after one second is 0.1ms
-1.
The ball's velocity is not going to stay at 0.1ms
-1 throughout the fall. It will pick up because of gravity. Let's say that gravity increases its velocity by 0.1ms
-1 at every second. Now imagine what happens after two seconds. Since gravity has increased the ball's velocity by 0.1ms
-1, it would have travelled at 0.2ms
-1. This means that from the first second to the second second, the ball has fallen 0.2m. Its position is now 99.9m - 0.2m = 99.7m.
In the third second, its velocity would increase again by 0.1ms
-1 to 0.3ms
-1. So its position is now 99.7m - 0.3m = 99.4m
This is exactly how we are going to program it. We will move the ball at very small time slices by changing its position and velocity at every iteration of a
forever block. Think of one iteration on the
forever block as a small time slice.
 |
Changing and keeping track of the position and velocity of the ball at every time slice |
Remember that we also need to increase its velocity (due to gravity) at each time slice. We separate the script for this to a different stack:
 |
Increasing velocity at each time slice |
And there we have it - a falling ball.
Step 3: Keep the ball afloat by blowing
The last thing we need to do is to make the ball float up as we blow into the microphone. The harder the blow, the louder is our puff and the faster the ball will float upwards. To do this, we will use the
loudness sensor. The
loudness sensor is found in the "Sensing" block group:
 |
loudness sensor |
The
loudness sensor has a Stage Monitor (or Watcher) that we can enable by ticking the checkbox next to it. We should be able to see the
loudness value on Stage once it's ticked:
 |
loudness Stage Monitor |
Connect a microphone and blow into it. The
loudness value is larger the stronger, or louder, you blow. So how shall we "blow the ball higher"? This is how:
 |
Script to move the higher the louder we blow |
All we do is change the velocity of the ball by a value factored by the loudness. We could merely change the
y velocity by the
loudness value, but the change would be too drastic - you could test it and see. So we pick a value, in this case 0.005, to multiply it by to adjust its effect.
Finally, we add a block to constantly turn the ball just so that it looks more natural and nice.
And that is all. Have fun!
Coming up: next week, we will learn how control a sprite with the keyboard. Til then!